レスポンシブにも対応した簡単にコーディングする3パターンのメニュー
フロントエンド
|
2017.07.15
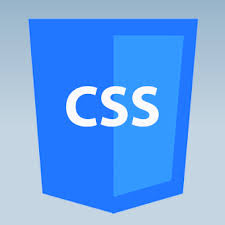
今回は同じような仕組みで、ダイアログ形式、ドロワー形式、アコーディオン形式のメニューを作成する方法をご紹介したいと思います。
共通のHTML部分
HTMLは同じ形式です。
メニューの調整は、cssとjqueryで調整します。
HTML
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<div class="wrapper"> <div id="header"> <div class="menu-btn" id="menu-btn"></div> </div> <div class="global-menu-wrap"> <div class="global-menu-inner"> <ul class="global-menu-link"> <li> <a href="#">リンク1</a> </li> <li> <a href="#">リンク2</a> </li> <li> <a href="#">リンク3</a> </li> <li> <a href="#">リンク4</a> </li> <li> <a href="#">リンク5</a> </li> </ul> </div> </div> </div> |
ダイアログ形式のメニュー
ダイアログ形式のメニューを作成する場合は、以下コードを使用してください。
css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
#header { height: 124px; position: relative; } #header .menu-btn { width: 60px; height: 60px; position: absolute; display: block; top: 35px; right: 20px; cursor: pointer; z-index: 9999 !important; } #header .menu-btn:before { content: ""; position: absolute; width: 26px; height: 6px; background: #666; left: 17px; transition: all 200ms; top: 22px; } #header .menu-btn:after { content: ""; position: absolute; width: 26px; height: 6px; background: #666; left: 17px; transition: all 200ms; top: 32px; } .show-global-menu { width: 100%; position: fixed; top: 0; left: 0; } .show-global-menu #header .menu-btn:before { -webkit-transform: rotate(45deg); transform: rotate(45deg); top: 27px; } .show-global-menu #header .menu-btn:after { -webkit-transform: rotate(-45deg); transform: rotate(-45deg); top: 27px; } .show-global-menu .global-menu-wrap { z-index: 10; opacity: 1; } .global-menu-wrap { position: fixed; width: 100%; height: 100vh; z-index: -1; background: rgba(255, 255, 255, 0.8); top: 0; left: 0; opacity: 0; transition: all 400ms; padding: 124px 0 0; } .global-menu-wrap .global-menu-inner { height: 100%; position: relative; text-align: center; } .global-menu-wrap .global-menu-inner .global-menu-link > li { display: block; margin-bottom: 20px; } .global-menu-wrap .global-menu-inner .global-menu-link > li > a { text-align: center; font-size: 28px; font-weight: bold; margin-bottom: 10px; color: #666; display: inline-block; position: relative; } .global-menu-wrap .global-menu-inner .global-menu-link > li > a:after { position: absolute; bottom: -5px; content: ''; width: 100%; height: 2px; background-color: #666; left: 0; } |
JQuery(JavaScript)
1 2 3 |
$('#menu-btn').click(function() { $('.wrapper').toggleClass('show-global-menu'); }); |
ドロワー形式のメニュー
ドロワー形式のメニューを作成する場合は、以下コードを使用してください。
css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 |
#header { position: relative; z-index: 20; height: 124px; } #header .menu-btn { width: 60px; height: 60px; position: absolute; display: block; top: 35px; right: 50px; cursor: pointer; z-index: 9999 !important; } #header .menu-btn:before { content: ""; position: absolute; width: 26px; height: 6px; background: #666; left: 17px; transition: all 200ms; top: 22px; } #header .menu-btn:after { content: ""; position: absolute; width: 26px; height: 6px; background: #666; left: 17px; transition: all 200ms; top: 32px; } .show-global-menu { width: 100%; position: fixed; top: 0; left: 0; } .show-global-menu #header .menu-btn:before { -webkit-transform: rotate(45deg); transform: rotate(45deg); top: 27px; } .show-global-menu #header .menu-btn:after { -webkit-transform: rotate(-45deg); transform: rotate(-45deg); top: 27px; } .show-global-menu .global-menu-wrap { z-index: 10; opacity: 1; } .show-global-menu .global-menu-wrap .global-menu-inner { right: 0; } .global-menu-wrap { position: fixed; width: 100%; height: 100vh; z-index: -1; background: rgba(0, 0, 0, 0.5); top: 0; left: 0; opacity: 0; transition: all 400ms; padding: 124px 0 0; } .global-menu-wrap .global-menu-inner { height: 100%; width: 300px; position: fixed; background: #fff; transition: all 400ms; right: -300px; z-index: 15; text-align: center; } .global-menu-wrap .global-menu-inner .global-menu-link > li { display: block; margin-bottom: 20px; } .global-menu-wrap .global-menu-inner .global-menu-link > li > a { text-align: center; font-size: 28px; font-weight: bold; margin-bottom: 10px; color: #666; display: inline-block; position: relative; } .global-menu-wrap .global-menu-inner .global-menu-link > li > a:after { position: absolute; bottom: -5px; content: ''; width: 100%; height: 2px; background-color: #666; left: 0; } |
JQuery(JavaScript)
1 2 3 |
$('#menu-btn').click(function() { $('.wrapper').toggleClass('show-global-menu'); }); |
アコーディオン形式のメニュー
アコーディオン形式のメニューを作成する場合は、以下コードを使用してください。
css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 |
#header { position: relative; z-index: 20; height: 124px; } #header .menu-btn { width: 60px; height: 60px; position: absolute; display: block; top: 35px; right: 50px; cursor: pointer; z-index: 9999 !important; } #header .menu-btn:before { content: ""; position: absolute; width: 26px; height: 6px; background: #666; left: 17px; transition: all 200ms; top: 22px; } #header .menu-btn:after { content: ""; position: absolute; width: 26px; height: 6px; background: #666; left: 17px; transition: all 200ms; top: 32px; } .show-global-menu { width: 100%; position: fixed; top: 0; left: 0; } .show-global-menu #header .menu-btn:before { -webkit-transform: rotate(45deg); transform: rotate(45deg); top: 27px; } .show-global-menu #header .menu-btn:after { -webkit-transform: rotate(-45deg); transform: rotate(-45deg); top: 27px; } .global-menu-wrap { position: absolute; display: none; z-index: 10; width: 100%; } .global-menu-wrap .global-menu-inner { height: 100%; background: #fff; text-align: center; } .global-menu-wrap .global-menu-inner .global-menu-link > li { display: block; margin-bottom: 20px; } .global-menu-wrap .global-menu-inner .global-menu-link > li > a { text-align: center; font-size: 28px; font-weight: bold; margin-bottom: 10px; color: #666; display: inline-block; position: relative; } .global-menu-wrap .global-menu-inner .global-menu-link > li > a:after { position: absolute; bottom: -5px; content: ''; width: 100%; height: 2px; background-color: #666; left: 0; } |
JQuery(JavaScript)
1 2 3 4 |
$('#menu-btn').click(function() { $('.wrapper').toggleClass('show-global-menu'); $('.global-menu-wrap').slideToggle(); }); |